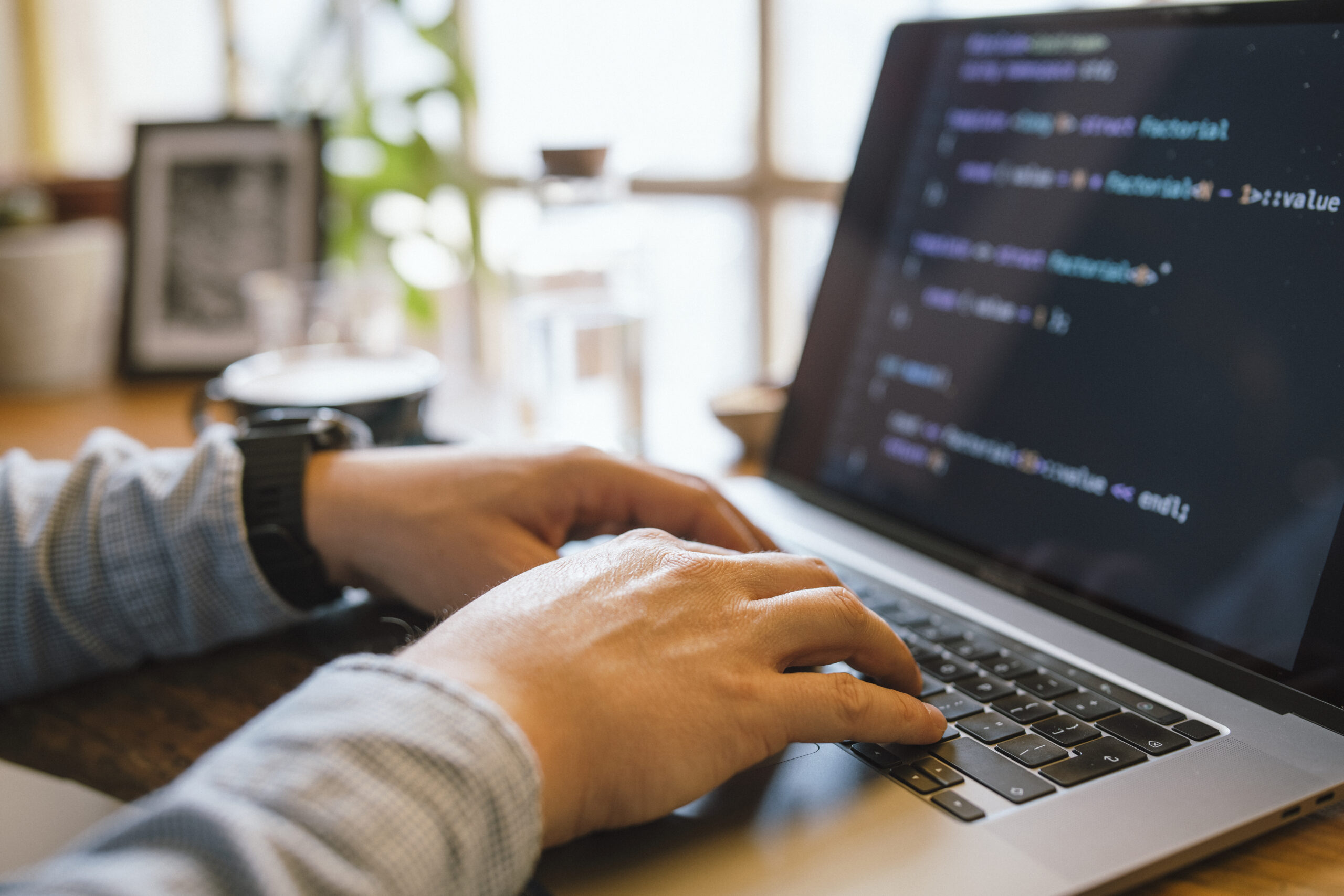
Debugging is one of the most important — nevertheless generally overlooked — abilities within a developer’s toolkit. It is not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Mastering to Assume methodically to unravel complications competently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging abilities can save hours of frustration and dramatically improve your efficiency. Here are quite a few procedures that can help builders degree up their debugging sport by me, Gustavo Woltmann.
Grasp Your Resources
One of many quickest ways builders can elevate their debugging techniques is by mastering the instruments they use daily. Whilst writing code is one Element of progress, being aware of the best way to interact with it proficiently in the course of execution is equally significant. Present day improvement environments occur Outfitted with strong debugging capabilities — but quite a few builders only scratch the surface of what these instruments can do.
Choose, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools help you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, and even modify code to the fly. When utilized effectively, they Enable you to observe just how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, resources, and network tabs can switch aggravating UI difficulties into manageable duties.
For backend or process-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory administration. Learning these applications may have a steeper Understanding curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfortable with Variation control programs like Git to be familiar with code history, locate the exact second bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments usually means likely over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress natural environment to make sure that when concerns come up, you’re not dropped in the dead of night. The greater you already know your instruments, the greater time you can spend solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the Problem
One of the most critical — and infrequently forgotten — techniques in powerful debugging is reproducing the challenge. Right before leaping to the code or producing guesses, developers need to produce a reliable ecosystem or circumstance wherever the bug reliably appears. With out reproducibility, correcting a bug will become a match of probability, usually leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as possible. Check with queries like: What actions brought about the issue? Which ecosystem was it in — enhancement, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the exact ailments below which the bug takes place.
After you’ve gathered adequate information, try to recreate the challenge in your local setting. This could indicate inputting the same knowledge, simulating similar person interactions, or mimicking program states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the edge scenarios or state transitions concerned. These checks not only support expose the condition and also prevent regressions Later on.
In some cases, the issue could possibly be ecosystem-particular — it would occur only on specified functioning systems, browsers, or below distinct configurations. Applying tools like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a state of mind. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now halfway to fixing it. By using a reproducible circumstance, You may use your debugging resources a lot more properly, exam likely fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Read and Recognize the Error Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Instead of seeing them as annoying interruptions, builders should learn to deal with mistake messages as direct communications from your method. They typically inform you what precisely transpired, wherever it took place, and from time to time even why it took place — if you know the way to interpret them.
Start out by looking at the concept very carefully and in complete. Lots of builders, particularly when beneath time strain, glance at the first line and promptly commence creating assumptions. But further inside the mistake stack or logs may possibly lie the genuine root lead to. Don’t just copy and paste error messages into search engines — browse and have an understanding of them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Will it level to a selected file and line variety? What module or function activated it? These questions can information your investigation and stage you toward the dependable code.
It’s also practical to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and learning to recognize these can greatly accelerate your debugging system.
Some mistakes are obscure or generic, As well as in People instances, it’s important to examine the context through which the error transpired. Test associated log entries, input values, and recent adjustments during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede larger sized issues and provide hints about likely bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint problems more rapidly, lower debugging time, and become a much more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When employed correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move with the code line by line.
A great logging technique starts with understanding what to log and at what level. Common logging levels involve DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details in the course of improvement, INFO for general situations (like effective start-ups), Alert for likely concerns that don’t break the applying, Mistake for genuine troubles, and Lethal if the program can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. An excessive amount of logging can obscure important messages and decelerate your procedure. Target crucial activities, state variations, enter/output values, and significant determination points within your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re In particular beneficial in output environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the long run, wise logging is about stability and clarity. Which has a effectively-considered-out logging strategy, you may reduce the time it requires to identify concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not simply a technological job—it's a method of investigation. To successfully discover and fix bugs, developers ought to method the process just like a detective resolving a secret. This attitude can help stop working complicated troubles into workable pieces and adhere to clues logically to uncover the basis induce.
Begin by collecting proof. Consider the indications of the trouble: mistake messages, incorrect output, or efficiency difficulties. Identical to a detective surveys against the law scene, collect just as much relevant information as you are able to without having leaping to conclusions. Use logs, exam conditions, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Inquire your self: What might be causing this actions? Have any variations a short while ago been designed to your codebase? Has this situation occurred ahead of beneath comparable circumstances? The intention should be to slim down prospects and determine potential culprits.
Then, exam your theories systematically. Try and recreate the situation in the controlled ecosystem. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Similar to a detective conducting interviews, request your code inquiries and let the effects direct you nearer to the truth.
Pay near interest to compact information. Bugs typically hide from the minimum envisioned areas—similar to a missing semicolon, an off-by-one error, or a race issue. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Short term fixes may cover the actual difficulty, just for it to resurface later.
Last of all, preserve notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term troubles and assist Other folks realize your reasoning.
By imagining like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed problems in sophisticated systems.
Compose Assessments
Crafting tests is one of the most effective strategies to transform your debugging skills and General advancement effectiveness. Tests not just aid catch bugs early but in addition function a security Internet that offers you assurance when earning changes for your codebase. A very well-analyzed software is much easier to debug as it means that you can pinpoint accurately where by and when a dilemma here takes place.
Get started with device checks, which concentrate on personal functions or modules. These little, isolated tests can rapidly reveal whether a specific bit of logic is Doing the job as envisioned. Every time a exam fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear soon after Formerly becoming preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These assist ensure that many areas of your software function together efficiently. They’re specifically useful for catching bugs that come about in intricate methods with various elements or providers interacting. If something breaks, your assessments can inform you which A part of the pipeline unsuccessful and below what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a aspect appropriately, you need to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a robust initial step. When the test fails persistently, you can give attention to correcting the bug and watch your examination move when The difficulty is resolved. This technique makes certain that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch additional bugs, a lot quicker and much more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is actually stepping away. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are far too near to the code for far too very long, cognitive exhaustion sets in. You might start overlooking obvious faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-solving. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your emphasis. Several developers report discovering the foundation of a challenge once they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during extended debugging periods. Sitting before a display, mentally trapped, is not simply unproductive and also draining. Stepping away allows you to return with renewed Electrical power plus a clearer state of mind. You might quickly recognize a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a very good guideline should be to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, nevertheless it basically contributes to a lot quicker and simpler debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a sensible approach. It presents your brain Room to breathe, increases your perspective, and allows you avoid the tunnel vision That always blocks your progress. Debugging can be a psychological puzzle, and rest is a component of fixing it.
Master From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It is really a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you anything precious for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring you several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit testing, code reviews, or logging? The answers often reveal blind places in your workflow or understanding and enable you to Create more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or popular issues—you can proactively prevent.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, assisting Other individuals avoid the similar concern boosts team effectiveness and cultivates a stronger Discovering lifestyle.
More importantly, viewing bugs as classes shifts your attitude from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. All things considered, a few of the most effective developers are not those who write best code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, more able developer because of it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.